Playlist Exporter beaTlet
A PlayListExporter is a component that beaTunes uses to export a playlist to a file. To see it in action, just right/control-click on a beaTunes playlist and choose Export Playlist... Built-in are exporters for the formats XSPF and M3U, but pretty much any format is possible. Perhaps you need to process detected keys in a spread sheet and therefore need to export CSV? Whatever you wantyou just need to write an appropriate exporter.
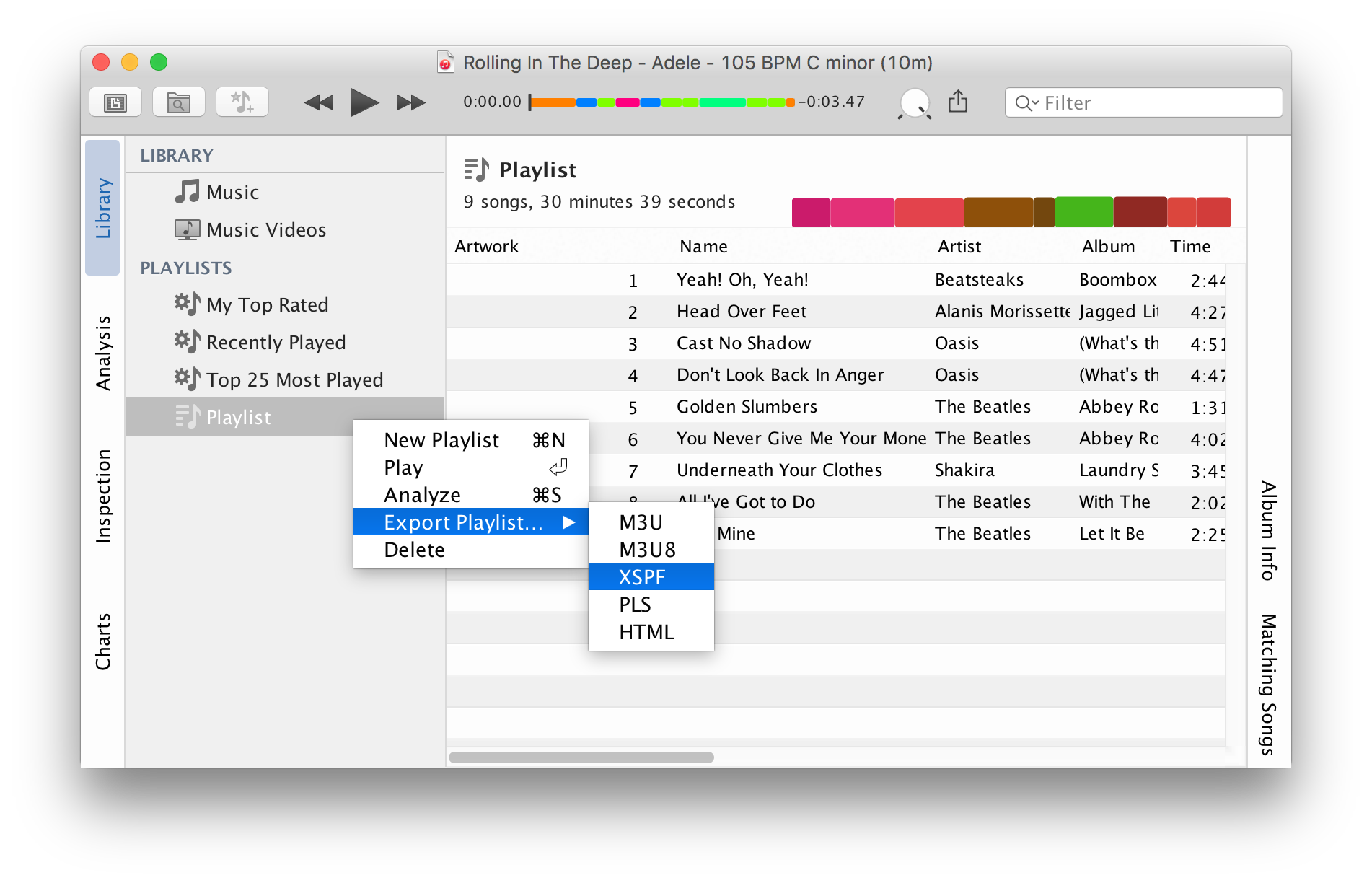
To give you an idea of how such an exporter can be written, here's one that simply lists all the songs in a playlist in HTML. As always, the code is simple and pretty self-explanatory:
require 'java' class HTMLPlayListExporter # The interface we need to implement. include Java::com.tagtraum.beatunes.library.PlayListExporter # Lowercase file extension (without the '.'). def getFileExtension() "html" end # Very short description for this exporter. # Starting with beaTunes 4.6, this method is optional. def getDescription() "HTML" end # Lets you provide an id for this exporter. # The id may be used for referring to an instance of this exporter # in persistent configuration files. def getId() "jruby.htmlplaylistexporter" end # Exports the given playlist to the given file. # # file to write to # playlist to export # useRelativePathsIfPossible flag for relative paths # progressListener that lets you report... well, progress def export(file, playList, useRelativePathsIfPossible, progressListener) f = nil begin f = File.new(file.toString, "w") f.write("<html>\n<body>\n<h1>#{playList.getName}</h1>\n<ol>\n") playList.getSongs.each{ |song| f.write("<li>#{song.getName}</li>\n") } f.write("</ol>\n</body>\n</html>\n") ensure if (!f.nil?) f.close end end end end
from com.tagtraum.beatunes.library import PlayListExporter # This example should use the codecs module, but due to # http://bugs.jython.org/issue1722 # this isn't possible. class HTMLPlayListExporter(PlayListExporter): # Lowercase file extension (without the '.'). def getFileExtension(self): return "html" # Due to http://bugs.jython.org/issue2403 the interface # default methods getId() and getDescription() # cannot be overridden. :-( # Exports the given playlist to the given file. # # file to write to # playlist to export # useRelativePathsIfPossible flag for relative paths # progressListener that lets you report... well, progress def export(self, file, playList, useRelativePathsIfPossible, progressListener): f = None try: f = open(file.toString(), 'w') f.write("<html>\n<body>\n<h1>%s</h1>\n<ol>\n" % (playList.getName().encode('UTF-8'))) for song in playList.getSongs(): f.write("<li>%s</li>\n" % (song.getName().encode('UTF-8'))) f.write("</ol>\n</body>\n</html>\n") finally: if (f is not None): f.close()
import java.io.File import com.tagtraum.core.ProgressListener import com.tagtraum.beatunes.library.PlayListExporter import com.tagtraum.beatunes.library.PlayList class HTMLPlayListExporter implements PlayListExporter { // Lowercase file extension (without the '.'). def String getFileExtension() { "html" } // Very short description for this exporter. // Starting with beaTunes 4.6, this method is optional. def String getDescription() { "HTML" } // Lets you provide an id for this exporter. // The id may be used for referring to an instance of this exporter // in persistent configuration files. def String getId() { "groovy.htmlplaylistexporter" } // Exports the given playlist to the given file. // // file to write to // playlist to export // useRelativePathsIfPossible flag for relative paths // progressListener that lets you report... well, progress def void export(File file, PlayList playList, boolean useRelativePathsIfPossible, ProgressListener progressListener) { file.withWriter { f -> f.writeLine("<html>\n<body>\n<h1>${playList.getName()}</h1>\n<ol>") playList.getSongs().each() { song -> f.writeLine("<li>${song.getName()}</li>") } f.writeLine("</ol>\n</body>\n</html>") } } }
/* * These type vars basically act as imports for Java classes. */ var PlayListExporter = Java.type("com.tagtraum.beatunes.library.PlayListExporter"); var FileWriter = Java.type("java.io.FileWriter"); var beatlet = new PlayListExporter() { /* Lowercase file extension (without the '.'). */ getFileExtension: function() { return "html"; }, /* * Very short description for this exporter. * Starting with beaTunes 4.6, this method is optional. */ getDescription: function() { return "HTML"; }, /* * Lets you provide an id for this exporter. * The id may be used for referring to an instance of this exporter * in persistent configuration files. */ getId: function() { return "Javascript.HTMLPlayListExporter"; }, /* * Exports the given playlist to the given file. * * file to write to * playlist to export * useRelativePathsIfPossible flag for relative paths * progressListener that lets you report... well, progress */ export: function(file, playList, useRelativePathsIfPossible, progressListener) { var writer = new FileWriter(file.toString()); try { writer.write("<html>\n<body>\n<h1>" + playList.getName() + "</h1>\n<ol>\n"); playList.getSongs().forEach(function(song) { writer.write("<li>" + song.getName() + "</li>\n"); }); writer.write("</ol>\n</body>\n</html>\n") } finally { writer.close(); } } } // Put "beatlet" into the last line, so that it is returned to beaTunes // when this script is eval'd. beatlet;
Want something a little more visual? Check out the song context view sample code.
Other beaTlet samples:
- Getting Started
- Context Action
- Library Batch Action
- Playlist Exporter
- Song Analysis Task
- beaTlet Plugin Descriptor
- Song Context View
- Song Property Analyzer
- Key Text Renderer
All sample beaTlets are also on
GitHub
.