Plugin Descriptors for beaTlets
Regular Java plugins for beaTunes have to communicate a number of things about themselves.
Things like their own version, the beaTunes version they require, change notes,
author, license etc. Because beaTlets aren't distributed through the central plugin repository,
they are not required to do so. However, if you plan on distributing your beaTlet in any way,
it's a good idea to declare a plugin descriptor. Plus, it looks nice in the plugins preferences pane
:-)
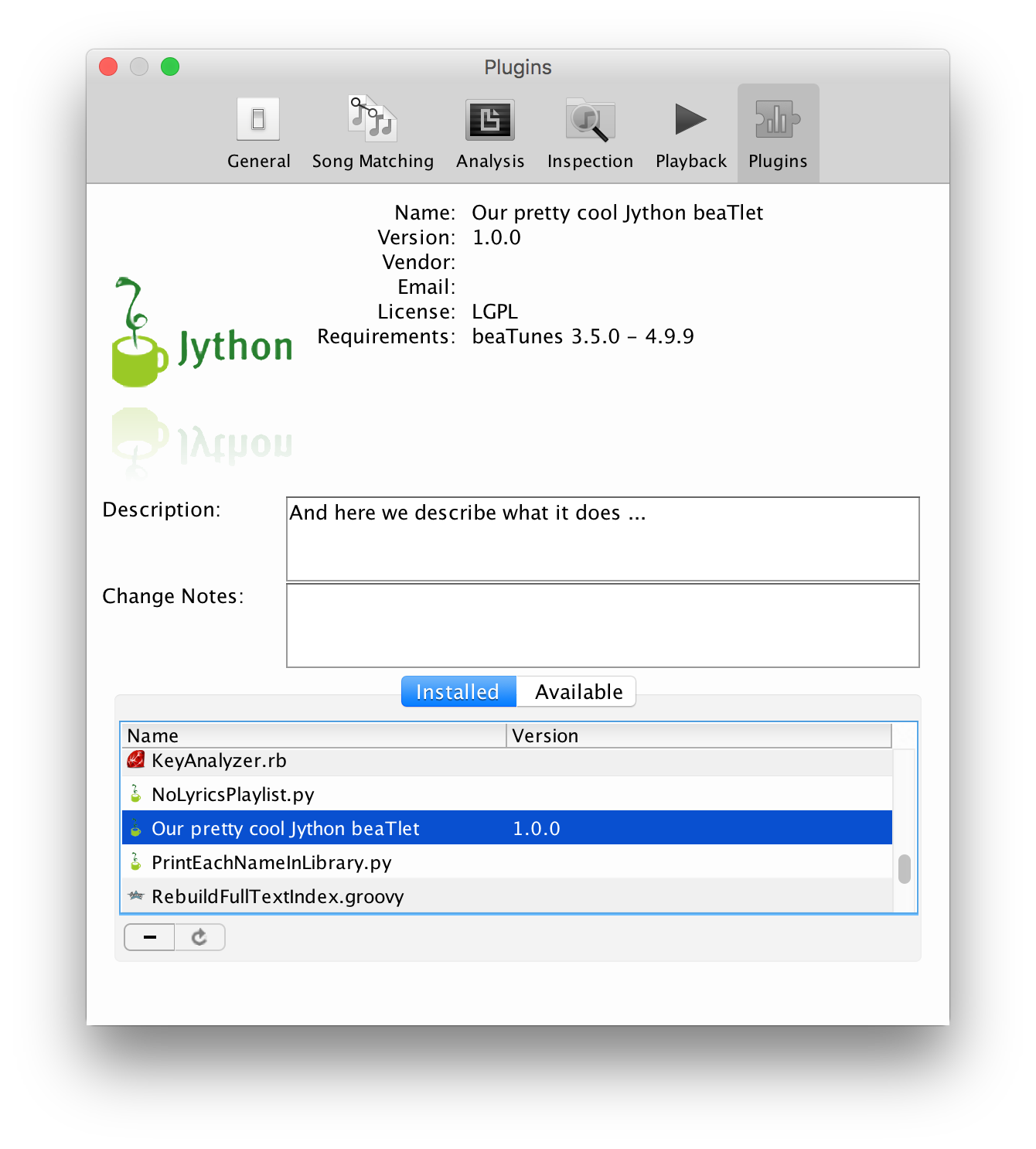
Unlike the declarative XML file used for Java plugins, beaTlets declare themselves programmatically. They should implement the com.tagtraum.core.app.Plugin interface. All this interface does, is provide a method to return a PluginDescriptor.
Here's how it's done:
from com.tagtraum.core.app import Plugin, PluginDescriptor, Version class PluginDescriptorDemo(Plugin): def getPluginDescriptor(self): pd = PluginDescriptor() pd.setId("com.yourdomain.someid.py") pd.setName("Our pretty cool Jython beaTlet") pd.setDescription("And here we describe what it does ...") pd.setLicenseName("LGPL") pd.setVersion(Version("1.0.0")) pd.setMinBeaTunesVersion(Version("3.5.0")) pd.setMaxBeaTunesVersion(Version("4.9.9")) # etc. return pd
import com.tagtraum.core.app.* class PluginDescriptorDemo implements Plugin { def PluginDescriptor getPluginDescriptor() { PluginDescriptor pd = new PluginDescriptor() pd.setId("com.yourdomain.someid.groovy") pd.setName("Our pretty cool Groovy beaTlet") pd.setDescription("And here we describe what it does ...") pd.setLicenseName("LGPL") pd.setVersion(new Version("1.0.0")) pd.setMinBeaTunesVersion(new Version("3.5.0")) pd.setMaxBeaTunesVersion(new Version("4.9.9")) // etc. return pd } }
require 'java' java_import com.tagtraum.core.app.Plugin java_import com.tagtraum.core.app.PluginDescriptor java_import com.tagtraum.core.app.Version class PluginDescriptorDemo include Java::com.tagtraum.core.app.Plugin def getPluginDescriptor() pd = PluginDescriptor.new() pd.setId("com.yourdomain.someid.rb") pd.setName("Our pretty cool JRuby beaTlet") pd.setDescription("And here we describe what it does ...") pd.setLicenseName("LGPL") pd.setVersion(Version.new("1.0.0")) pd.setMinBeaTunesVersion(Version.new("3.5.0")) pd.setMaxBeaTunesVersion(Version.new("4.9.9")) # etc. return pd end end
var Plugin = Java.type("com.tagtraum.core.app.Plugin"); var PluginDescriptor = Java.type("com.tagtraum.core.app.PluginDescriptor"); var Version = Java.type("com.tagtraum.core.app.Version"); var beatlet = new Plugin() { getPluginDescriptor: function() { var pd = new PluginDescriptor(); pd.setId("com.yourdomain.someid.rb"); pd.setName("Our pretty cool JavaScript beaTlet"); pd.setDescription("And here we describe what it does ..."); pd.setLicenseName("LGPL"); pd.setVersion(new Version("1.0.0")); pd.setMinBeaTunesVersion(new Version("3.5.0")); pd.setMaxBeaTunesVersion(new Version("4.9.9")); // etc. return pd; } } beatlet;
Other beaTlet samples:
- Getting Started
- Context Action
- Library Batch Action
- Playlist Exporter
- Song Analysis Task
- Song Context View
- Song Property Analyzer
- Key Text Renderer
All sample beaTlets are also on
GitHub
.